projects /Acadex | Desktop Web App
Project Overview and Features
The 2022 Conference on Empirical Methods in Natural Language Processing (EMNLP2022) released their list of accepted works in early November. Unfortunately, the list of 1381 accepted submissions was published in the form of an Excel file which was difficult to peruse and filter.
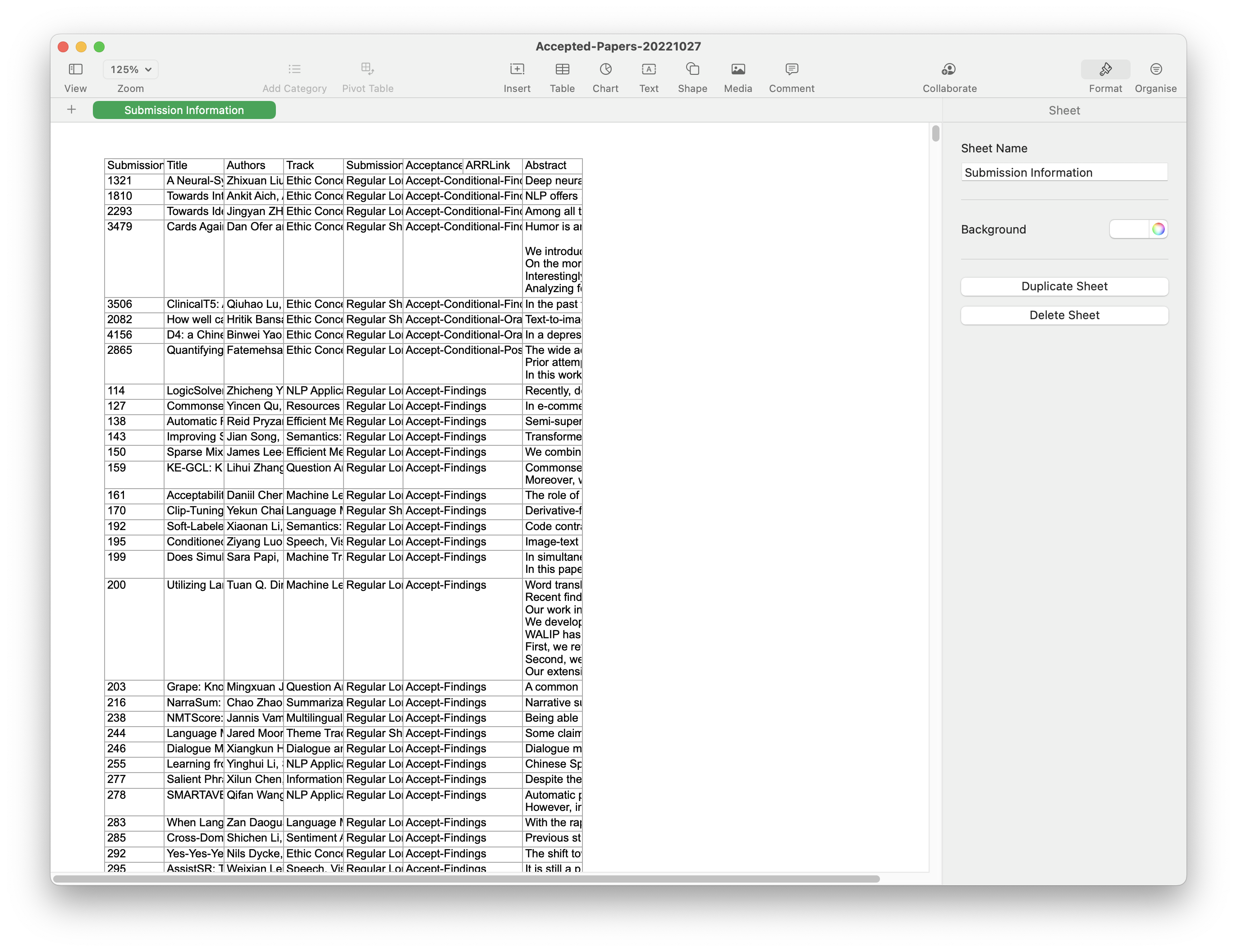
I created a simple desktop web app to display the contents of this excel file, and also implemented features to support searching and filtering of submissions, as well as 'bookmarking' submissions of particular interest to users.
The abstracts of each submission was displayed in a popover modal.
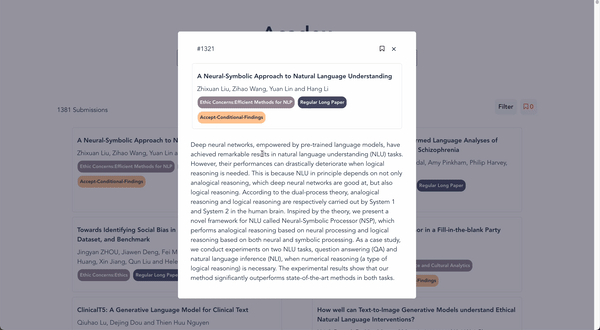
Searching and filtering were implemented through the use of simple React useState
hooks, and basic JS array filtering methods.
Search by Title or Author:
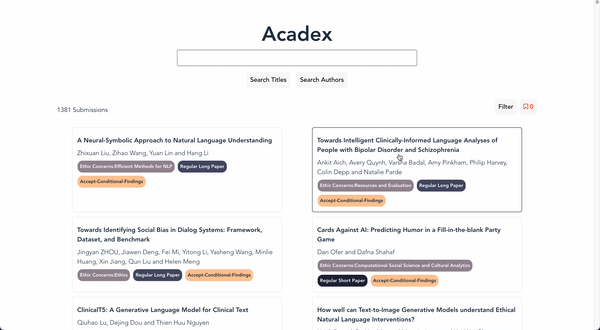
Multi-Filter submission by track, submission type, and/or acceptance status:
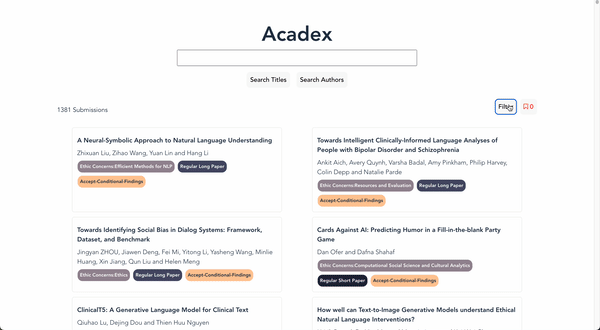
Code for handling multiple optional filters:
function handleFilter() {
let tracks: string[] = [];
let types: string[] = [];
let status: string[] = [];
if (selectedTrack.length) {
tracks = selectedTrack.map((el: { label: string; value: string }) => {
return el.value;
});
}
if (selectedSubType.length) {
types = selectedSubType.map((el: { label: string; value: string }) => {
return el.value;
});
}
if (selectedAccStatus.length) {
status = selectedAccStatus.map((el: { label: string; value: string }) => {
return el.value;
});
}
setData((prev) => {
return prev.filter((el: dataProps) => {
let hasTracks =
el.Track && tracks.length ? tracks.includes(el.Track) : true;
let hasTypes =
el.Submission_Type && types.length
? types.includes(el.Submission_Type)
: true;
let hasStatus =
el.Acceptance_Status && status.length
? status.includes(el.Acceptance_Status)
: true;
let result = hasTracks && hasTypes && hasStatus;
return result;
});
});
}
Persisting bookmarked submissions in LocalStorage:
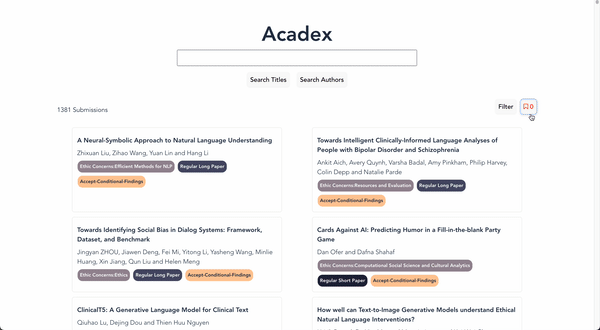